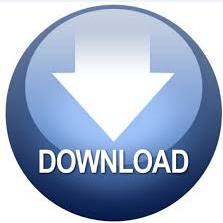

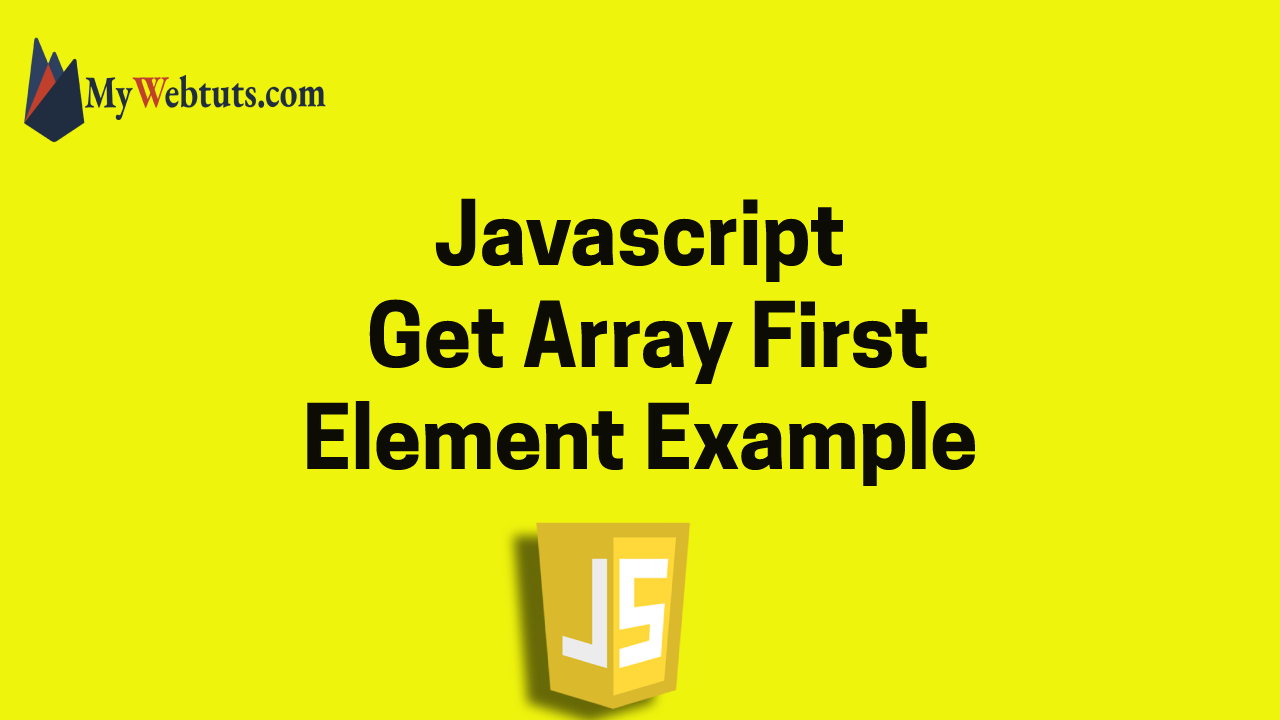
If you want to check if any element is present or not in array, then you can just simply check the index of that element in array. let shop = console.log(shop.indexOf('Amazon', 1)) // -1 If we specify a position, then it will start looking for element from that position till end of the array.
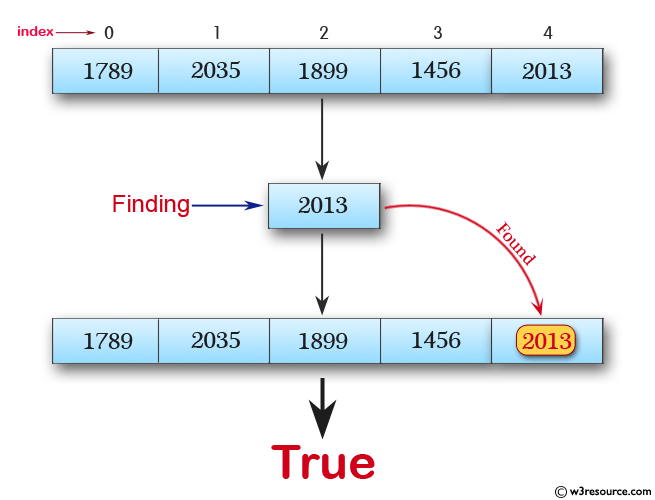
This method accepts an optional parameter which specifies the position to start the search for element. let shop = console.log(shop.indexOf('Myntra')) // 3 console.log(shop.indexOf('Flipkart')) // 1 console.log(shop.indexOf('amazon')) // -1 If no element is matched then it returns -1. This method returns the index of first occurrence of matched element in array. let teams = console.log(teams.includes('KKR', 2)) // false The indexOf() method let teams = console.log(teams.includes('KKR')) // true console.log(teams.includes('rcb')) // false If the specified element is present in array then it returns true else false. This method checks if a specified element is present or not in an array and returns a boolean value. let score = let index = score.findIndex(val => val > 60) // returns the index of first element that passed the condition console.log(index) // 1 The includes() method If no match found in the array then findIndex() returns -1. The findIndex() method works the same way as find() method except find() returns the first matched element and findIndex() returns the index of the first matched element. This method also takes a function argument which returns true or false. The Array findIndex() method returns the index of first matched element in array that satisfies a condition. let score = let value = score.find(val => val > 60) // returns the first element that passed the condition console.log(value) // 73 The findIndex() method If no element passed the condition then find() method will return undefined. If the function returns true for any element then that element will be returned by the find() method and it stops checking further for rest of the elements. The find() method executes this function for each element of array. The find() method takes a function as argument which returns true or false based on some condition. The Array find() method returns the first matched element in array that satisfies a condition. The various methods are find, findIndex, includes, indexOf, lastIndexOf and loops ( for, filter, map). We will be discussing about 6 ways to find in array. whether we are searching for element or its position in the array. But we can always choose the method that satisfies our search criteria i.e. There are various methods available to find elements or their positions in Array.
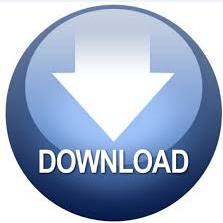